【文章內容使用 Gemini 1.5 Pro 自動產生】
在 Flutter 中處理網頁手勢
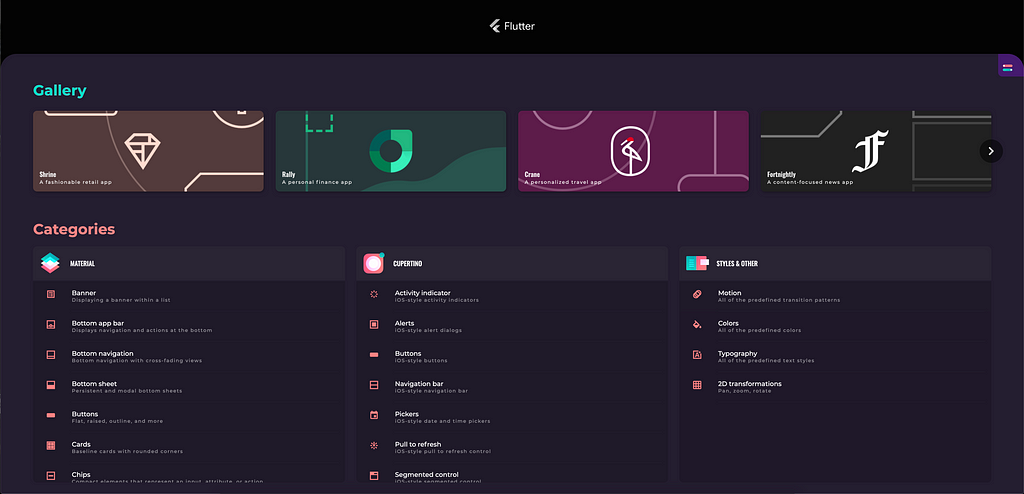
Flutter 發佈了網頁支援,讓您可以建立動態網站。有了 Flutter 網頁、MacOS、Linux 和 Windows 作業系統(甚至在連接到滑鼠、鍵盤或其他週邊設備的行動裝置上),您現在可以存取平台特定行為,包括焦點支援、鍵盤輸入、自訂滑鼠游標和懸停輸入。但是,您如何在 Flutter 中使用這些平台行為呢?
本文說明如何使用 FocusableActionDetector
Widget,它處理鍵盤輸入、焦點輸入、懸停輸入和自訂滑鼠游標。
如何使用 FocusableActionDetector
Widget
FocusableActionDetector
Widget 允許您處理幾個網頁特定動作,包括:
- 鍵盤輸入
- 焦點輸入
- 懸停輸入
- 自訂滑鼠游標
在 DartPad #1 範例中查看 FocusableActionDetector
的實際應用。
**注意:**本文使用了兩個 DartPad 範例,儘管每個範例都有多個連結。[DartPad #1](https://dartpad.dev/d16345202d0e26d40fe14904657dc24a) 展示了 `FocusableActionDetector` 範例,而 [DartPad #2](https://dartpad.dev/5544c57e20454fe212e3ec5cf10b1f0a) 展示了 `FocusableActionDetector` 的懸停和焦點支援。
鍵盤輸入
Flutter 允許您定義自訂動作來處理鍵盤輸入。假設您想要有一個隱藏的選單,或者您的應用程式必須處理大量的自訂鍵盤輸入。使用 FocusableActionDetector
Widget 很容易做到這一點。
首先,加入以下匯入:
1 | import 'package:flutter/services.dart'; |
接下來,從調用 FocusableActionDetector
的類別中,初始化兩個私有變數。這些變數將鍵盤輸入映射到所需的動作:
1 | Map<LogicalKeySet, Intent> _shortcutMap; |
1 | Map<Type, Action<Intent>> _actionMap; |
定義一個 FocusableActionDetector
Widget。請注意,自訂鍵盤輸入僅在子 Widget 獲得焦點時才會啟用:
1 | FocusableActionDetector( |
此類別將列舉和鍵盤輸入類型映射到 Intent
類別。以下設定是必要的,因為 actions
和 shortcuts
參數需要一個繼承自 Intent
的類別:
1 | class _ShowSecretMessageIntent extends Intent { |
在 initState
方法中,初始化之前定義的映射:
1 | void initState() { |
動作處理程序將鍵盤輸入連結到應用程式中的特定函數。每次按下字元時,都會在應用程式的主要部分加入一個展開的容器。但在您的應用程式中,您可以將其用於更具創意目的:
1 | void _actionHandler(_ShowSecretMessageIntent intent) { |
焦點輸入
FocusableActionDetector
Widget 允許您在指定 Widget 獲得焦點時執行動作呼叫。
焦點樹允許您使用 Tab 鍵在應用程式中瀏覽。這讓有視力障礙的人能夠瀏覽您的網站。
FocusableActionDetector
子 Widget 被選為初始焦點,當其作用域中的其他節點目前沒有焦點時。如果您希望您的自訂鍵盤輸入在子 Widget 是否有焦點都不受影響的情況下運作,則將 autofocus
參數變數設定為 true
很重要。這是因為,預設情況下,此 FocusableActionDetector
Widget 將不會獲得焦點,因此您將無法使用自訂鍵盤輸入:
1 | FocusableActionDetector( |
假設您希望子 Widget 在獲得焦點時具有不同的顏色,以識別此 Widget 目前是否有焦點。如果您需要在 Widget 獲得焦點時設定自訂動作,請使用 onShowFocusHighlight
屬性。
DartPad #2 範例展示了如何使用 onShowFocusHighlight
屬性。
焦點是一個進階主題。透過閱讀 Focus 類別的 API 文件,您可以進一步了解焦點。
懸停輸入
FocusableActionDetector
Widget 允許您處理懸停輸入。這樣一來,每當游標懸停在特定 Widget 上時,就會發生自訂動作。與 onShowFocusHighlight
屬性類似,onShowHoverHighlight
屬性讓您能夠在滑鼠游標懸停在特定 Widget 上時建立自訂動作。
查看 DartPad #2 範例,它展示了如何實作自訂懸停和焦點動作。
在 DartPad #2 範例中,每當滑鼠懸停在 Widget 上時,它就會更改 Widget 的顏色。如果您使用 Tab 鍵在應用程式中瀏覽,您會注意到,與目前被懸停的 Widget 相比,獲得焦點的 Widget 會對 Widget 應用不同的陰影。
這意味著您可以在 Widget 獲得焦點或滑鼠游標懸停在該 Widget 上時,執行不同的自訂動作。如果您將滑鼠懸停在同一個 Widget 上並使它獲得焦點,您就可以看出來。該 Widget 會同時對該 Widget 應用兩個陰影。若要了解更多資訊,請查看 FocusableActionDetector 類別的 API 文件。
自訂滑鼠游標
FocusableActionDetector
Widget 讓您能夠實作自訂滑鼠游標。例如,如果您希望使用者知道特定 Widget 是可拖曳的,更改滑鼠游標是一個很好的指示,表示您可以執行此操作。FocusableActionDetector
Widget 具有 mouseCursor
參數。
1 | mouseCursor: SystemMouseCursors.grabbing |
DartPad #1 範例展示了 Flutter 中不同類型的滑鼠游標。點擊應用程式列中的任何圖示以嘗試使用。有九種不同的系統預設滑鼠游標:
SystemMouseCursors.basic
SystemMouseCursors.text
SystemMouseCursors.click
SystemMouseCursors.forbidden
SystemMouseCursors.grab
SystemMouseCursors.grabbing
SystemMouseCursors.horizontalDoubleArrow
(未在穩定版本中)SystemMouseCursors.verticalDoubleArrow
(未在穩定版本中)SystemMouseCursors.none
結語
在 Flutter 中,您可以存取網頁特定 API。FocusableActionDetector
Widget 讓您能夠在 Flutter 中處理網頁特定功能。若要進一步了解 Flutter 網頁,請查看 Medium 文章 Handling 404: Page not found error in Flutter。
關於作者:Jose 最近從大學畢業,現在在 Material 工作,這是一個幫助團隊構建高品質數位體驗的設計系統。Jose 的團隊維護 Flutter 的 material 函式庫。若要了解更多資訊,請訪問 Jose 在 GitHub、LinkedIn、YouTube 和 Instagram 上的頁面。
在 Flutter 中處理網頁手勢 最初發佈在 Flutter 上的 Medium,人們在那裡透過突出顯示和回應這個故事來繼續討論。